Rotor model visualizations¶
Rotor models are responsible for averaging ambient background quantities over the rotor disc, yielding rotor equivalent ambient results.
Note that in foxes wake effects are averaged by partial wakes models, not by rotor models.
In [1]:
import foxes
import matplotlib.pyplot as plt
mbook = foxes.ModelBook()
CentreRotor¶
The simplest rotor model consists of a single point located at the rotor centre. For spatially uniform ambient states, that’s all you need - but beware of chosing this as a partial wakes model, since for wakes rotor averaging is always crucial.
In [2]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["centre"])
ax = o.get_point_figure()
plt.show()
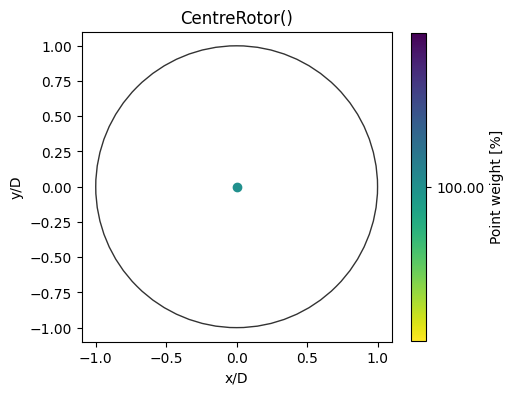
GridRotor¶
Grid rotors are based on regular two dimensional grids with non-uniform weights. The weights represent the overlap of the area element that is associated with each rotor point and the rotor disc.
Concrete grid rotors with N x N
points can be constructed as GridRotor(n=N)
. Note that points with zero weights will be removed from the point list. These models are available in the default model book under the name template grid<n2>
, where n2
represents N x N
.
If you prefer to switch off the weights, i.e., use equal weights for all points of the regular grid, simply set reduce=False
as an argument of the constructor. Such models are available in the default model book under the name template grid<n2>_raw
, where n2
represents N x N
.
grid4¶
In [3]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid4"])
ax = o.get_point_figure()
plt.show()
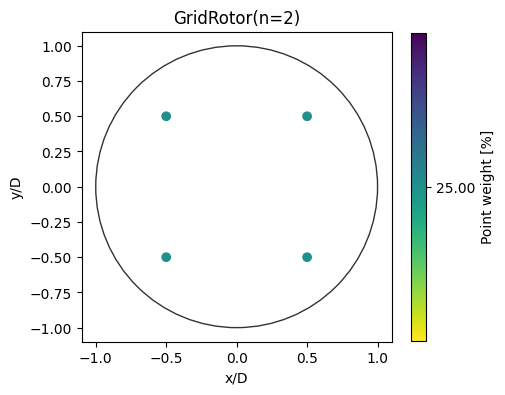
grid9¶
In [4]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid9"])
ax = o.get_point_figure()
plt.show()
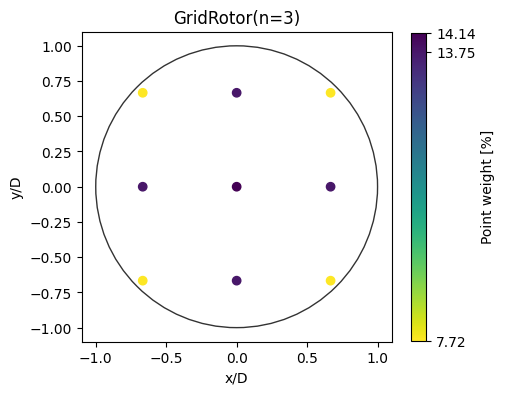
grid16¶
In [5]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid16"])
ax = o.get_point_figure()
plt.show()
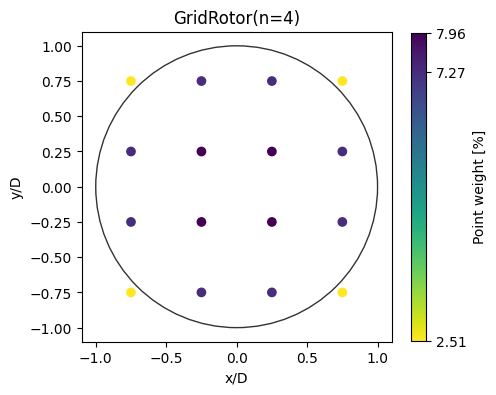
grid25¶
In [6]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid25"])
ax = o.get_point_figure()
plt.show()
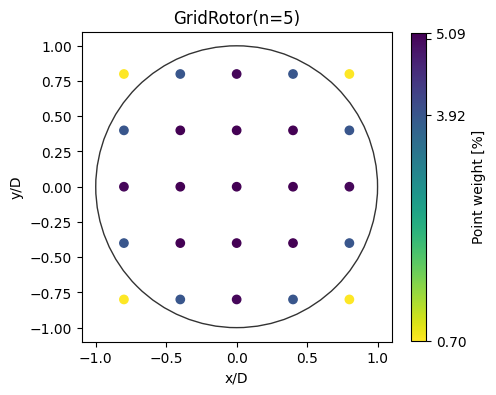
grid25_raw¶
In [7]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid25_raw"])
ax = o.get_point_figure()
plt.show()
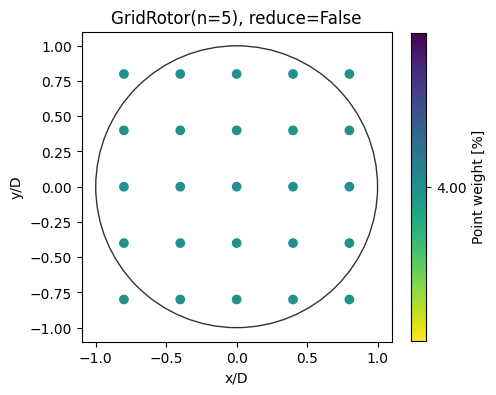
grid36¶
In [8]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid36"])
ax = o.get_point_figure()
plt.show()
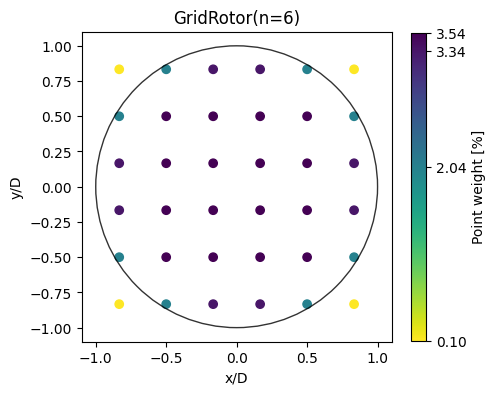
grid100¶
In [9]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid100"])
ax = o.get_point_figure()
plt.show()
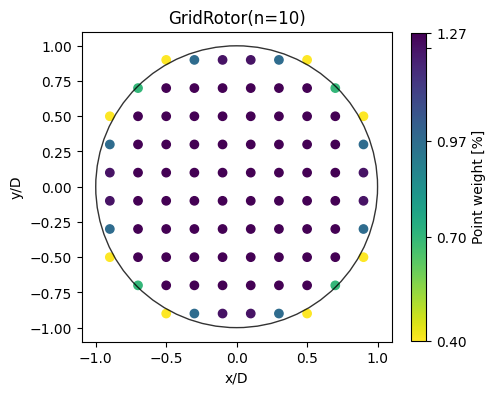
grid400¶
In [10]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["grid400"])
ax = o.get_point_figure()
plt.show()
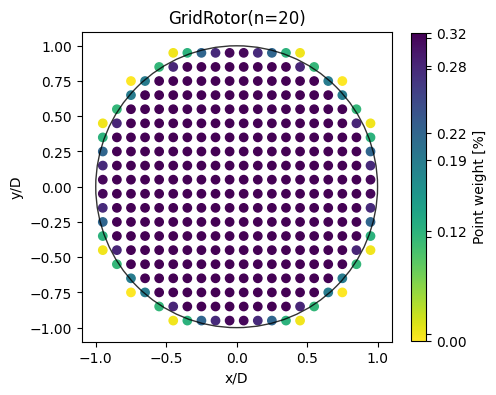
LevelRotor¶
Level rotors consist of a single vertical line through the rotor centre. Each point represents a vertical slice of the rotor disc. These rotors are intended to be used in combination with ambient states that vary in vertical direction only (height dependent data).
Never combine this rotor model with partial wakes rotor_points
, since for wake averaging, the rotor points simply don’t make much sense.
A level rotor with N
levels can be constructed as LevelRotor(n=N)
(add reduce=False
if uniform weights should be used). The default model book contains level rotors with N
levels under the name templates level<N>
and level<N>_raw
for reduce=True
and reduce=False
, respectively.
level2¶
In [11]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level2"])
ax = o.get_point_figure()
plt.show()
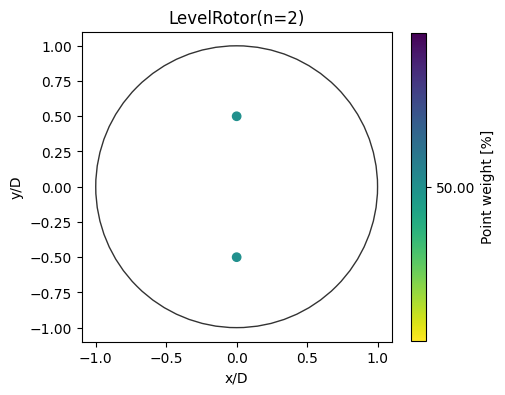
level3¶
In [12]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level3"])
ax = o.get_point_figure()
plt.show()
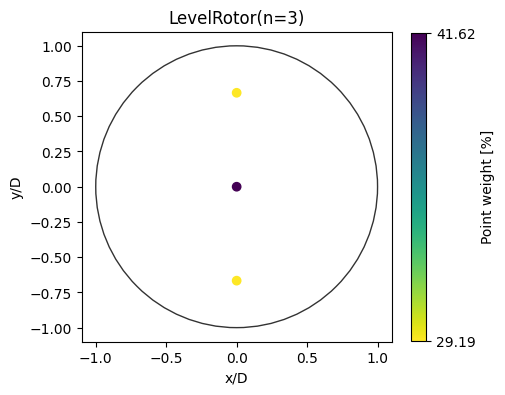
level5¶
In [13]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level5"])
ax = o.get_point_figure()
plt.show()
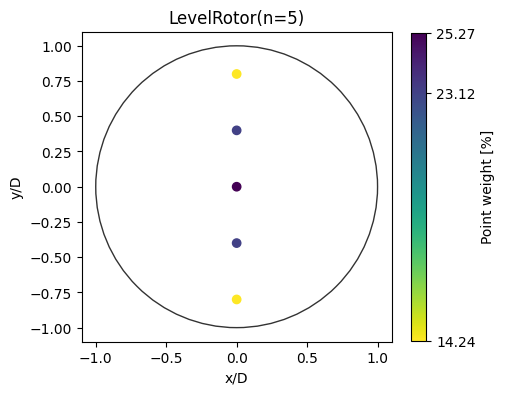
level5_raw¶
In [14]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level5_raw"])
ax = o.get_point_figure()
plt.show()
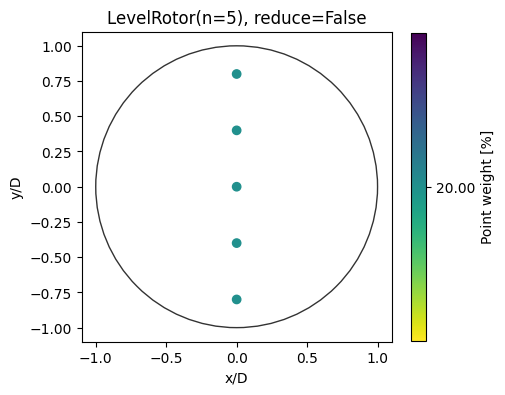
level10¶
In [15]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level10"])
ax = o.get_point_figure()
plt.show()
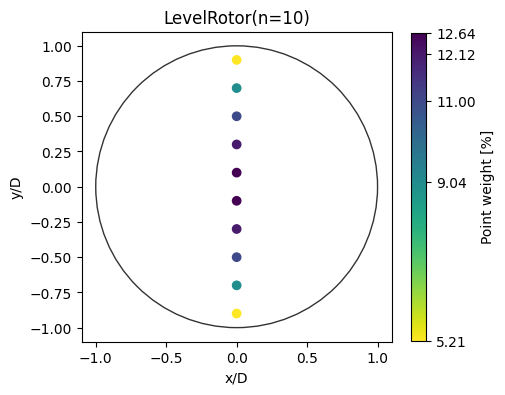
level20¶
In [16]:
o = foxes.output.RotorPointPlot(mbook.rotor_models["level20"])
ax = o.get_point_figure()
plt.show()
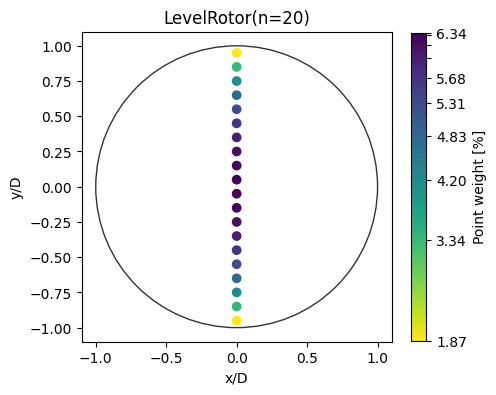